top of page
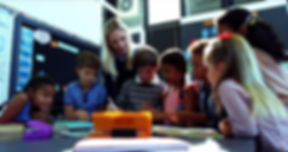
게시판 게시물
minjukim3p
2021년 3월 21일
In 소스 코드 제출
/* import java.util.*; public class Main { String name; int year; int inch; Main(String n, int y, int i) { name=n; year=y; inch=i; } void show() { System.out.printf(name+"에서 만든 "+year+"년형"+inch+"인치 TV"); } public static void main(String[] args) { Main myTV=new Main("LG",2017,32); myTV.show(); } } */ /* import java.util.*; class Grade{ int math; int science; int english; Grade(int math,int science,int english) { this.math=math; this.science=science; this.english=english; } int average() { return (math+science+english)/3; } } public class Main{ public static void main(String[] args) { Scanner t=new Scanner(System.in); System.out.printf("수학, 과학, 영어 순으로 3개의 점수 입력>>"); int math = t.nextInt(); int science = t.nextInt(); int english = t.nextInt(); Grade me = new Grade(math, science, english); System.out.println("평균은 " + me.average()); } } */ /* import java.util.*; class song{ String title; String artist; int year; String country; song(String title, String artist, int year, String country) { this.title=title; this.artist=artist; this.year=year; this.country=country; } void show() { System.out.printf(year+"년 "+country+"국적의 "+artist+"가 부른 "+title); } } public class Main{ public static void main(String[] args) { song a= new song("Dancing Queen","ABBA",1987,"스웨덴"); a.show(); } } */ /* import java.util.*; class rectangle{ int x; int y; int width; int height; rectangle(int x,int y,int width,int height){ this.x=x; this.y=y; this.width=width; this.height=height; } int square(){ return(width*height); } void show() { System.out.printf("(" +x+ "," +y+ ")" +"에서 크기가"+ width+"x"+height+"인 사각형"); } boolean contains(rectangle r) { } public class Main{ public static void main(String[] args) { rectangle r = new rectangle(2,2,8,7); rectangle s = new rectangle(5,5,6,6); rectangle t = new rectangle(1,1,10,10); r.show(); System.out.println("s의 면적은 " + s.square()); if(t.contains(r)) { System.out.println("t는 r을 포함합니다."); } if(t.contains(s)) { System.out.println("t는 s을 포함합니다."); } } } */ import java.util.*; class Day{ private String work; public void set(String work) { this.work = work; } public String get() { return work; } public void show() { if(work == null) System.out.println("없습니다"); else System.out.println(work+"입니다."); } } class MonthSchedule{ Day[] d; MonthSchedule (int n) { d=new Day[n]; for(int i=0;i<n;i++) { d[i]=new Day(); } } void run() { Scanner t=new Scanner(System.in); System.out.println("이번달 스케쥴 관리 프로그램"); while(true) { System.out.print("할일(입력:1, 보기:2, 끝내기:3) >>"); int n = t.nextInt(); if(n==1) input(); else if(n==2) view(); else { finish(); return ; } } } void input() { Scanner t=new Scanner(System.in); System.out.print("날짜(1~30)?"); int day =t.nextInt(); System.out.print("할일(빈칸없이입력)?"); String w=t.next(); d[day].set(w); } void view() { Scanner t=new Scanner(System.in); System.out.print("날짜(1~30)?"); int day =t.nextInt(); System.out.print(day+"일의 할 일은 "); d[day].show(); } void finish() { System.out.println("프로그램을 종료합니다."); } public static void main(String[] args) { MonthSchedule april=new MonthSchedule(30); april.run(); } }
0
0
2
minjukim3p
2021년 3월 14일
In 소스 코드 제출
/* import java.util.*; class Rectangle { int x; int y; int width; int height; Rectangle(int x,int y,int width,int height){ this.x=x; this.y=y; this.width=width; this.height=height; } int square() { return width*height; } void show() { System.out.println("("+x+","+y+")"+"에서 크기가"+width+"x"+height+"인 사각형"); } boolean contains(Rectangle r) { if(x<r.x && y<r.y && x+height>r.x+r.height &&y+width>r.y+r.width) return true; else return false; } } public class Main { public static void main(String[] args) { Rectangle r = new Rectangle(2,2,8,7); Rectangle s = new Rectangle(5,5,6,6); Rectangle t = new Rectangle(1,1,10,10); r.show(); System.out.println("s의 면적은 " + s.square()); if(t.contains(r)) { System.out.println("t는 r을 포함합니다."); } if(t.contains(s)) { System.out.println("t는 s을 포함합니다."); } } } */ /* import java.util.Scanner; class Circle{ private double x,y; private int radius; Circle(double x,double y,int radius) { this.x=x; this.y=y; this.radius=radius; } public void show() { System.out.println("("+x+","+y+")"+radius); } } public class Main{ public static void main(String[] args) { Scanner s=new Scanner(System.in); Circle c[]=new Circle[3]; for(int i=0;i<c.length;i++) { System.out.print("x, y, radius >>"); double x=s.nextDouble(); double y=s.nextDouble(); int radius=s.nextInt(); c[i]=new Circle(x, y, radius); } for(int i=0;i<c.length;i++) c[i].show(); s.close(); } } */ /* import java.util.Scanner; class Circle{ private double x,y; private int radius; Circle(double x,double y,int radius) { this.x=x; this.y=y; this.radius=radius; } public int size() { return radius; } public void show() { System.out.println("("+x+","+y+")"+radius); } } public class Main{ public static void main(String[] args) { Scanner s=new Scanner(System.in); Circle c[]=new Circle[3]; for(int i=0;i<c.length;i++) { System.out.print("x, y, radius >>"); double x=s.nextDouble(); double y=s.nextDouble(); int radius=s.nextInt(); c[i]=new Circle(x, y, radius); } for(int i=0;i<c.length;i++) { c[i].size(); if(c[0].size()<c[2].size()&&c[2].size()<c[1].size()) { System.out.println("가장면적이 큰 원은"+c[1].size()); } if(c[2].size()<c[1].size()&&c[1].size()<c[0].size()) { System.out.println("가장면적이 큰 원은"+c[0].size()); } if(c[1].size()<c[0].size()&&c[0].size()<c[2].size()) { System.out.println("가장면적이 큰 원은"+c[2].size()); } } s.close(); } } */
0
0
1
minjukim3p
2021년 3월 07일
In 소스 코드 제출
/* import java.util.*; public class Main { String name; int year; int inch; Main(String n, int y, int i) { name=n; year=y; inch=i; } void show() { System.out.printf(name+"에서 만든 "+year+"년형"+inch+"인치 TV"); } public static void main(String[] args) { Main myTV=new Main("LG",2017,32); myTV.show(); } } */ /* import java.util.*; class Grade{ int math; int science; int english; Grade(int math,int science,int english) { this.math=math; this.science=science; this.english=english; } int average() { return (math+science+english)/3; } } public class Main{ public static void main(String[] args) { Scanner t=new Scanner(System.in); System.out.printf("수학, 과학, 영어 순으로 3개의 점수 입력>>"); int math = t.nextInt(); int science = t.nextInt(); int english = t.nextInt(); Grade me = new Grade(math, science, english); System.out.println("평균은 " + me.average()); } } */ /* import java.util.*; class song{ String title; String artist; int year; String country; song(String title, String artist, int year, String country) { this.title=title; this.artist=artist; this.year=year; this.country=country; } void show() { System.out.printf(year+"년 "+country+"국적의 "+artist+"가 부른 "+title); } } public class Main{ public static void main(String[] args) { song a= new song("Dancing Queen","ABBA",1987,"스웨덴"); a.show(); } } */ import java.util.*; class rectangle{ int x; int y; int width; int height; rectangle(int x,int y,int width,int height){ this.x=x; this.y=y; this.width=width; this.height=height; } int square(){ return(width*height); } void show() { System.out.printf("(" +x+ "," +y+ ")" +"에서 크기가"+ width+"x"+height+"인 사각형"); } boolean contains(rectangle r) { } public class Main{ public static void main(String[] args) { rectangle r = new rectangle(2,2,8,7); rectangle s = new rectangle(5,5,6,6); rectangle t = new rectangle(1,1,10,10); r.show(); System.out.println("s의 면적은 " + s.square()); if(t.contains(r)) { System.out.println("t는 r을 포함합니다."); } if(t.contains(s)) { System.out.println("t는 s을 포함합니다."); } } }
0
0
2
minjukim3p
2021년 1월 28일
In 소스 코드 제출
//import java.util.*; // //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int [][]arr=new int[100][100]; // int x1; // int y1; // int x2; // int y2; // int cnt=0; // for(int i=0;i<4;i++) { // x1=t.nextInt(); // y1=t.nextInt(); // x2=t.nextInt(); // y2=t.nextInt(); // for(int n=x1;n<x2;n++) { // for(int m=y1;m<y2;m++) { // if(x1<=n&&n<=x2) { // arr[n][m]=1; // } // else if(y1<=m&&m<=y2) { // arr[n][m]=1; // } // } // // } // // } // for(int k=0;k<100;k++) { // for(int l=0;l<100;l++) { // if(arr[k][l]==1) { // cnt++; // } // } // // } // System.out.println(cnt); // } //} /* import java.util.*; class calcu { int a, b; calcu() { a = 0; b = 0; } calcu(int a, int b) { this.a = a; this.b = b; display('+'); display('-'); display('*'); display('/'); } int sum(int a, int b) { return a+b; } int sum(int a, float b) { return a-(int)b; } int sum(float a, int b) { return (int)a*b; } int sum() { return a/b; } void display(char oper) { switch(oper) { case '+': System.out.println(); break; case '-': System.out.println(); break; case '*'; System.out.println(a); } } /* int sum(int a, int b) { return a+b; } int sum(char a, char b) { return a-b; } float sum(float a, float b) { return a*b; } double sum(double a,double b) { return a/b; } void display(int a, int b) { System.out.println(a +"+"+b+"="+sum((int)a,(int) b)); } void display(char a,char b) { System.out.println(a+"-"+b+"="+sum((char) a,(char) b)); } void display(float a,float b) { System.out.println(a+"*"+b+"="+sum((float) a,(float) b)); } void display(double a,double b) { System.out.println(a+"/"+b+"="+sum((double) a,(double) b)); } */ /* } public class Main { public static void main(String[] args) { Scanner t = new Scanner(System.in); int a = t.nextInt(); int b = t.nextInt(); calcu c = new calcu(a, b); } } */ /* public class Main{ int radius; String name; public Main() { radius = 1; name=""; } public Main(int r,String n) { radius = r; name = n; } public double getArea() { return 3.14*radius*radius; } public static void main(String[] args) { Main pizza = new Main(10, "피자"); double area =pizza.getArea(); System.out.println(pizza.name+"의 면적은"+area); Main donut = new Main(); donut.name ="도넛피자"; area =donut.getArea(); System.out.println(donut.name+"의 면적은"+ area); } } */ /* public class Main{ String title; String author; public Main(String t) { title=t; author = "작자미상"; } public Main(String t,String a) { title=t; author = a; } public static void main(String[] args) { Main LittlePrince= new Main("어린왕자","생텍쥐페리"); Main LoveStory=new Main("춘향전"); System.out.println(LittlePrince.title + " " + LittlePrince.author); System.out.println(LoveStory.title + " " + LoveStory.author); } } */ /* public class Main{ String title; String author; void show() {System.out.println(title + " " + author); } public Main() { this("", ""); System.out.println("생성자 호출됨"); } public Main(String title) { this(title,"작자미상"); } public Main(String title,String author) { this.title =title; this.author=author; } public static void main(String[] args) { Main []aaa; Main LittlePrince= new Main("어린왕자", "생텍쥐페리"); Main LoveStory=new Main("춘향전"); Main emptyBook= new Main(); LoveStory.show(); } } */ /* public class Main{ String LG; int year; int inch; Main() { this("", 0, 0); } Main(String LG, int year, int inch){ this.LG = LG; this.year=2017; this.inch=32; } void show() { //System.out.println("LG" + "year" + "inch"); System.out.printf("%s %d %d", LG,year,inch ); } public static void main(String[] args) { Main myTV=new Main("LG", 2017, 32); myTV.show(); } } */ /* import java.util.*; class Parents { int a, b, c; Parents() { a = 10; b = 20; c = 30; } void talk() { System.out.println("IM YOUR FATHER"); } } class Children extends Parents { Children() { a = 100; b = 200; c = 300; } void talk() { System.out.println("IM YOUR SUN"); } } public class Main { public static void main(String[] args) { Children c = new Children(); Parents p = new Parents(); p.talk(); c.talk(); } } */ import java.util.*; class Student{ String name[],grade[]; int age[]; Student(){} void info() { //System.out.printf("%s %d %s",name,age,grade); } } class Academy extends Student { Academy(){} Academy(int peopleCnt) { name = new String[peopleCnt]; grade = new String[peopleCnt]; age = new int[peopleCnt]; } void inputData(String []name, String []grade, int []age) { for(int i=0; i<name.length; i++) { this.name[i] = name[i]; } for(int i=0;i<grade.length;i++) { this.grade[i]=grade[i]; } for(int i=0;i<age.length;i++) { this.age[i]=age[i]; } } void findStudent(String name){ for(int i=0; i<this.name.length; i++) { if(name.equals(this.name[i])) { System.out.printf("%s %s %d",this.name[i], grade[i],age[i]); } } // System.out.printf("%d %s",age,grade); } } public class Main{ public static void main(String[] args) { String []name = {"Ike", "Poll","a","b","c" }; String []grade = {"A","B","C","D","E"}; int []age = {1,2,3,4,5}; Academy a = new Academy(10); a.inputData(name, grade ,age); a.findStudent("Ike"); } }
0
0
8
minjukim3p
2021년 1월 21일
In 소스 코드 제출
//import java.util.*; // //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int [][]arr=new int[100][100]; // int x1; // int y1; // int x2; // int y2; // int cnt=0; // for(int i=0;i<4;i++) { // x1=t.nextInt(); // y1=t.nextInt(); // x2=t.nextInt(); // y2=t.nextInt(); // for(int n=x1;n<x2;n++) { // for(int m=y1;m<y2;m++) { // if(x1<=n&&n<=x2) { // arr[n][m]=1; // } // else if(y1<=m&&m<=y2) { // arr[n][m]=1; // } // } // // } // // } // for(int k=0;k<100;k++) { // for(int l=0;l<100;l++) { // if(arr[k][l]==1) { // cnt++; // } // } // // } // System.out.println(cnt); // } //} /* import java.util.*; class calcu { int a, b; calcu() { a = 0; b = 0; } calcu(int a, int b) { this.a = a; this.b = b; display('+'); display('-'); display('*'); display('/'); } int sum(int a, int b) { return a+b; } int sum(int a, float b) { return a-(int)b; } int sum(float a, int b) { return (int)a*b; } int sum() { return a/b; } void display(char oper) { switch(oper) { case '+': System.out.println(); break; case '-': System.out.println(); break; case '*'; System.out.println(a); } } /* int sum(int a, int b) { return a+b; } int sum(char a, char b) { return a-b; } float sum(float a, float b) { return a*b; } double sum(double a,double b) { return a/b; } void display(int a, int b) { System.out.println(a +"+"+b+"="+sum((int)a,(int) b)); } void display(char a,char b) { System.out.println(a+"-"+b+"="+sum((char) a,(char) b)); } void display(float a,float b) { System.out.println(a+"*"+b+"="+sum((float) a,(float) b)); } void display(double a,double b) { System.out.println(a+"/"+b+"="+sum((double) a,(double) b)); } */ /* } public class Main { public static void main(String[] args) { Scanner t = new Scanner(System.in); int a = t.nextInt(); int b = t.nextInt(); calcu c = new calcu(a, b); } } */ /* public class Main{ int radius; String name; public Main() { radius = 1; name=""; } public Main(int r,String n) { radius = r; name = n; } public double getArea() { return 3.14*radius*radius; } public static void main(String[] args) { Main pizza = new Main(10, "피자"); double area =pizza.getArea(); System.out.println(pizza.name+"의 면적은"+area); Main donut = new Main(); donut.name ="도넛피자"; area =donut.getArea(); System.out.println(donut.name+"의 면적은"+ area); } } */ /* public class Main{ String title; String author; public Main(String t) { title=t; author = "작자미상"; } public Main(String t,String a) { title=t; author = a; } public static void main(String[] args) { Main LittlePrince= new Main("어린왕자","생텍쥐페리"); Main LoveStory=new Main("춘향전"); System.out.println(LittlePrince.title + " " + LittlePrince.author); System.out.println(LoveStory.title + " " + LoveStory.author); } } */ /* public class Main{ String title; String author; void show() {System.out.println(title + " " + author); } public Main() { this("", ""); System.out.println("생성자 호출됨"); } public Main(String title) { this(title,"작자미상"); } public Main(String title,String author) { this.title =title; this.author=author; } public static void main(String[] args) { Main []aaa; Main LittlePrince= new Main("어린왕자", "생텍쥐페리"); Main LoveStory=new Main("춘향전"); Main emptyBook= new Main(); LoveStory.show(); } } */ public class Main{ String LG; int year; int inch; Main() { this("", 0, 0); } Main(String LG, int year, int inch){ this.LG = LG; this.year=2017; this.inch=32; } void show() { //System.out.println("LG" + "year" + "inch"); System.out.printf("%s %d %d", LG,year,inch ); } public static void main(String[] args) { Main myTV=new Main("LG", 2017, 32); myTV.show(); } }
0
0
1
minjukim3p
2021년 1월 14일
In 소스 코드 제출
import java.util.Arrays; import java.util.Scanner; /* import java.util.*; public class Main { public static void main(String[] args) { Scanner t = new Scanner(System.in); int a = t.nextInt(); System.out.print(a + "\n"+"HELLO"); System.out.println("234234"); } } */ /* public class Main { public static void main(String[] args) { int [][]arr; int n = 10; int m = 20; arr = new int[n][m]; String str = "asdasd"; for(int i=0; i<arr.length; i++) { for(int j=0; j<arr[i].length; j++) { } } } } */ //import java.util.*; // //public class Main{ // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // float double // System.out.println(a +"+"+b+"="+(a+b)); // System.out.printf("%d-%d=%d\n", a, b, a-b); // System.out.println(a + "*" +b +"="+ (a*b)); // System.out.println(a + "/"+ b+"="+ (a/b)); // } //} /* public class Main{ public static void main(String[] args) { Scanner t = new Scanner(System.in); int n; int m; int [][]arr = new int[10][10]; for(n=0;n<10;n++) { for(m=0;m<10;m++) { arr[n][m]=t.nextInt(); } } n=1; m=1; for(;;){ if(arr[n][m]==0){ arr[n][m]=9; if(arr[n][m+1]==0) { m++; } else if(arr[n][m+1]==1) { if(arr[n+1][m]==0) { n++; } else if(arr[n+1][m]==1) { break; } else if(arr[n+1][m]==2) { arr[n+1][m]=9; break; } } else { arr[n][m+1]=9; break; } } else if(arr[n][m]==2) { arr[n][m]=9; break; } } for(int i=0;i<10;i++) { for(int j=0;j<10;j++) { System.out.print( arr[i][j] +" "); } System.out.print("\n"); } } } */ /* import java.util.*; class food { String name; int cal; int value; void getName() { System.out.println("This food is " + name); } } public class Main { public static void main(String[] args) { Scanner t = new Scanner(System.in); food geupsic[] = new food[100]; for(int i=0; i<geupsic.length; i++) { geupsic[i] = new food(); geupsic[i].name = "Sogogi Gukbab"; } food Apple = new food(); Apple.name = "SAGWA"; Apple.cal = 35; Apple.value = 1250; Apple.getName(); } } */ /* 학생 5명의 정보를 받아 모두 출력하는 프로그램을 짜시오 * 정보는 이름, 국어점수, 수학점수, 영어점수 * * 모두 출력하는 기능은 stdInfoPrint() 메서드를 이용하시오. * * * */ /* import java.util.*; class student{ String name; int rnrdj; int tngkr; int duddj; void stdInfoPrint() { System.out.println(name+rnrdj+tngkr+duddj); } } public class Main{ public static void main(String[] args) { Scanner t = new Scanner(System.in); student st[]=new student[5]; for(int i=0; i<st.length;i++) { st[i] = new student(); st[i].name = t.next(); st[i].rnrdj=t.nextInt(); st[i].tngkr=t.nextInt(); st[i].duddj=t.nextInt(); } for(int j=0;j<4;j++) { st[j].stdInfoPrint(); } } } */ class sub { String name; int n; // contructor - initailize // method overloading sub() { name = "IKE"; } sub(String name) { this.name = name; show(); } void show() { } // } public class Main { public static void main(String[] args) { sub s = new sub("ASD"); System.out.println(s.name); } }
0
0
2
minjukim3p
2021년 1월 07일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ /* #include <stdio.h> int main() { int arr[10]={1,2,3,4,5,6,7,8,9,10},left,right,mid,k; scanf("%d", &k); left = 0; right = 9; mid = (left+right)/2; while(1) { if(k==arr[mid]) { printf("%d",k); break; } else { if(k>arr[mid]) { left = mid; mid = (left+right)/2; } else if(k<arr[mid]){ right = mid; mid =(left+ right)/2; } } if(left==right&&arr[mid]!=k){ printf("-1"); break; } } } */ /* 1 2 3 4 5 6 7 8 9 0 */ // 유네#3604 /* #include <stdio.h> int main() { int arr[1000000],q[1000000],left,right,mid,k,i,j,y,x,l; scanf("%d",&y); for(i=0; i<y; i++) { scanf("%d",&arr[i]); } scanf("%d",&x); for(j=0; j<x; j++) { scanf("%d",&q[j]); } for(l=0; l<x; l++) { left=0; right=y-1; mid=(left+right)/2; while(1) { if(q[l]==arr[mid]) { printf("%d ",mid+1); break; } else { if(q[l]>arr[mid]) { left = mid+1; mid = (left+right)/2; } else if(q[l]<arr[mid]) { right = mid; mid =(left+ right)/2; } } if(left==right&&arr[mid]!=q[l]) { printf("-1 "); break; } } } */
0
0
1
minjukim3p
2020년 12월 03일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ /* #include <stdio.h> #include "DlinkedList.h" int whoisPrecede(int d1,int d2) { if(d1<d2){ return 0; } else{ return 1; } } int main(void) */ #include <stdio.h> #include <stdlib.h> #include <malloc.h> typedef struct _node { int data; struct _node *next; } Node; int main(void) { Node *head=NULL; Node *tail=NULL; Node *cur=NULL; Node *newNode=NULL; Node *DummyNode=NULL; int readData; while(1) { scanf("%d", &readData); if(readData<1) { break; } newNode=(Node*)malloc(sizeof(Node)); newNode->data=readData; if(head==NULL) { head=newNode; newNode->next = NULL; tail=newNode; } else { cur=head; while( cur->next!=NULL && cur->next->data<readData) { cur=cur->next; } newNode->next = cur->next; cur->next = newNode; } printf("=======\n"); cur=head; printf("%d ",cur->data); while(cur->next!=NULL) { cur=cur->next; printf("%d ",cur->data); } printf("\n=======\n"); } /* printf("\n"); if(head==NULL) { printf("---------------------------"); } else { cur=head; printf("%d ",cur->data); while(cur->next!=NULL) { cur=cur->next; printf("%d ",cur->data); } } printf("\n"); if(head==NULL) { return 0; } else { Node* delNode=head; Node*delNextNode=head->next; printf("%d",head->data); free(delNode); while(delNextNode!=NULL) { delNode=delNextNode; delNextNode=delNextNode->next; printf("%d\n",delNode->data); free(delNode); } }*/ return 0; }
0
0
1
minjukim3p
2020년 11월 26일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ #include <stdio.h> #include <stdlib.h> #include <malloc.h> typedef struct _node { int data; struct _node *next; }Node; int main(void) { Node *head=NULL; Node *tail=NULL; Node *cur=NULL; Node *newNode=NULL; int readData; while(1) { scanf("%d", &readData); if(readData<1){ break; } newNode=(Node*)malloc(sizeof(Node)); newNode->data=readData; newNode->next=NULL; if(head==NULL){ head=newNode; } else{ tail->next=newNode; } tail=newNode; } printf("\n"); if(head==NULL){ printf("---------------------------"); } else{ cur=head; printf("%d ",cur->data); while(cur->next!=NULL){ cur=cur->next; printf("%d ",cur->data); } } printf("\n"); if(head==NULL){ return 0; } else { Node* delNode=head; Node*delNextNode=head->next; printf("%d",head->data); free(delNode); while(delNextNode!=NULL) { delNode=delNextNode; delNextNode=delNextNode->next; printf("%d\n",delNode->data); free(delNode); } } return 0; } /* #include<stdio.h> int cpr(int *pArr) { *pArr = 100; } int main() { int a; int *pA; int arr[100]={10,20,30}; int *pArr = arr; pA = &a; printf("%p\n", &a); printf("%p\n", pA); printf("%p\n", &arr[0]); printf("%p\n", arr); printf("%d %d %d\n", *arr, *(arr+1), *arr+1); cpr(pArr); printf("%d %d %d\n", *arr, *(arr+1), *arr+1); } */
0
0
2
minjukim3p
2020년 11월 19일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ /* #include <stdio.h> int main() { int n,i,j,a[18][18]={0}; scanf("%d",&n); for(i=1;i<17;i++){ for(j=1;j<17;j++){ scanf("%1d",&a[i][j]); } } for(i=0;i<17;i++){ for(j=0;j<17;j++){ if(a[i][j]==0){ if(a[i+1][j+1]==3||a[i+1][j]==3||a[i+1][j-1]==3||a[i][j-1]==3||a[i-1][j-1]==3||a[i-1][j]==3||a[i-1][j+1]==3){ printf("1"); } else if(a[i+1][j+1]!=3||a[i+1][j]!=3||a[i+1][j-1]!=3||a[i][j-1]!=3||a[i-1][j-1]!=3||a[i-1][j]!=3||a[i-1][j+1]!=3){ printf("0"); } } } } } */ /* #include <stdio.h> void rec(int n) { int a[18][18]; if(a[18][18]==3){ printf("1"); return 0; } } int main() */ #include<stdio.h> int map[18][18] = {0}; int sX, sY, fX, fY; int flag; int p = 5; void move(int x, int y) { if(map[x][y] == 3){ flag = 1; return ; } map[x][y] = 1; if(map[x-1][y]==0||map[x-1][y]==3) { move(x-1, y); } if(map[x][y+1]==0||map[x][y+1]==3) { move(x,y+1); } if(map[x+1][y]==0||map[x+1][y]==3) { move(x+1,y); } if(map[x][y-1]==0||map[x][y-1]==3) { move(x,y-1); } } int calcu() { flag = 0; move(sX, sY); return flag; } int main() { int i, j, k, n; for(k=0; k<10; k++) { scanf("%d", &n); for(i=1; i<17; i++) { for(j=1; j<17; j++) { scanf("%1d", &map[i][j]); if(map[i][j] == 2) { sX = i; sY = j; } if(map[i][j] == 3) { fX = i; fY = j; } } } printf("#%d %d\n", n, calcu()); } }
0
0
2
minjukim3p
2020년 11월 14일
In 소스 코드 제출
/* #include <stdio.h> struct w { int a; int b; }; int main() { struct w k[101]={0};; int n,p=0,l=0,i,j; scanf("%d",&n); for(i=0; i<n; i++) { scanf("%d %d",&k[i].a,&k[i].b); } for(i=0; i<n; i++) { for(j=0; j<n-1; j++) { if(k[j].a > k[j+1].a) { p = k[j].a; k[j].a = k[j+1].a; k[j+1].a = p; l = k[j].b; k[j].b = k[j+1].b; k[j+1].b = l; } } } for(i=0; i<n; i++) { printf("%d %d\n",k[i].a,k[i].b); } return 0; } #include<stdio.h> struct w { int a; int b; }; int main() { struct w x[201]={0};; int n,i,j; scanf("%d",&n); for(i=1;i<=n;i++) { scanf("%d",&x[i].a); x[i].b=1; } for(i=1;i<=n;i++) { for(j=1;j<=n;j++) { if(x[i].a>x[j].a) { x[j].b++; } } } for(i=1;i<=n;i++) { printf("%d %d\n",x[i].a,x[i].b); } } */ #include<stdio.h> struct w { char a; int b; int c; }; int main() { struct w k[101]; int a,b,n,i,j,d,e,g; scanf("%d",&n); for(i=1;i<=n;i++) { scanf("%c %d %d %d",&a,&b,&e,&g); } for(i=1;i<=n;i++) { for(j=1;j<=n;j++) { if(k[i].b<k[j].b) { } if(e[i]>e[j]) { k[j].f++; } if(g[i]>g[j]) { k[j].h++; } }
0
0
2
minjukim3p
2020년 11월 12일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ /* #include <stdio.h> int main() { int t,n,i,l[100][1000],k[100][1001],j,m=1,h; scanf("%d",&t); for(h=0; h<t; h++) { scanf("%d",&n); for(i=0; i<n; i++) { scanf(" %c %d",&k[h][i],&l[h][i]); } } for(h=0; h<t; h++) { m=1; printf("#%d\n",h+1); for(i=0; i<n; i++) { for(j=1; j<=l[h][i]; j++) { printf("%c",k[h][i]); if(m%10==0&&m!=0) { printf("\n"); } m++; } } printf("\n"); } } 10 3 A 10 B 7 C 5 4 B 20 C 19 E 18 R 17 5 Y 10 J 3 G 5 Z 7 A 11 6 Q 16 P 5 O 1 I 2 B 3 V 4 7 C 6 Z 20 M 10 J 10 G 10 H 8 T 2 8 R 1 Y 3 U 9 L 15 Z 14 N 11 X 8 W 7 10 S 4 D 12 H 3 A 16 O 9 X 5 V 1 C 5 H 10 W 14 1 U 14 2 I 6 K 2 10 S 4 D 12 H 13 A 16 O 19 X 15 V 11 C 15 H 11 W 13 */
0
0
1
minjukim3p
2020년 11월 05일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ #include <stdio.h> int main() { int n,i,y,m,d,k,a[10]; scanf("%d",&n); for(i=0; i<n; i++) { scanf("%d",&a[i]); } for(i=0; i<n; i++) { y=a[i]/10000; m=a[i]%10000/100; d=a[i]%10000%100; if(m>12||m==0) { printf("#%d -1\n",i+1); } else if(m==2&&d>28) { printf("#%d -1\n",i+1); } else if(m<8&&m%2==1&&d>31) { printf("#%d -1\n",i+1); } else if(m>8&&m%2==0&&d>31) { printf("#%d -1\n",i+1); } else if(m<8&&m%2==0&&d>30) { printf("#%d -1\n",i+1); } else if(m>8&&m%2==1&&d>30) { printf("#%d -1\n",i+1); } else { printf("#%d %04d/%02d/%02d\n",i+1,y,m,d); } } }
0
0
2
minjukim3p
2020년 10월 29일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ /* #include <stdio.h> int main() { int n,i,m,sum=0; scanf("%d",&n); for(i=0;i<1;i++){ m=n/10; sum+=m%10; sum+=n%10; sum+=m/10%10; sum+=m/10/10; } printf("%d\n",sum); } */ /* #include <stdio.h> #include <string.h> int main() { char str[1000]; int i; scanf("%s",str); for(i=0; i<strlen(str); i++) { if('A'<=str[i] && str[i]<='Z') { printf("%d ",str[i]-64); } } return 0; } */
0
0
2
minjukim3p
2020년 10월 22일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ /* #include <stdio.h> int grap[20][3]= {0}; int n; int calcu(int ct) int calcu1(int ct) { int sum=grap[0][ct]; int i, j, min=50000000;; int cot=-1; for(i=1; i<n; i++) { min=50000000; for(j=0; j<3; j++) { if(j!=ct&&min>grap[i][j]) { min=grap[i][j]; cot=j; } } sum+=min; ct=cot; cot=-1; } int sum1=grap[0][ct]; int k,l, min1=50000000;; int cot=-1; for(k=n; k>1; k--) { min1=50000000; for(l=3; l>0; l--) { if(l!=ct&&min>grap[k][l]) { min=grap[k][l]; cot=l; } } sum+=min; ct=cot; cot=-1; } return sum1; } int main() { int i; scanf("%d",&n); for(i=0; i<n; i++) { scanf("%d %d %d %d %d %d",&grap[i][0],&grap[i][1],&grap[i][2],&grap[k][1],&grap[k][2],&grap[k][0]); } if(calcu(1)>calcu(0)&&calcu(2)>calcu(0)&&calcu1(1)>calcu){ printf("%d",calcu(0)); } else if(calcu(0)>calcu(1)&&calcu(2)>calcu(1)){ printf("%d",calcu(1)); } else if(calcu(1)>calcu(2)&&calcu(0)>calcu(2)){ printf("%d",calcu(2)); } } */ /* #include <stdio.h> #include <string.h> int main() { char str[1000]; int i; scanf("%s",str); for(i=0;i<strlen(str);i++){ if('a'<=str[i]&&str[i]<='z'){ printf("%c",str[i]-32); } else{ printf("%c",str[i]); } } return 0; } */ /* #include <stdio.h> int main() { int a,i; scanf("%d",&a); for(i=0;i<a;i++){ printf("%c",35); } } */ #include <stdio.h> int main() { int a[10000],i,sum; for(i=0;i<=a;i++){ } }
0
0
1
minjukim3p
2020년 10월 15일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ /* #include <stdio.h> int main() { int n,min,i,j,sum1=0,sum2=0,sum3=0,sum4=0,sum5=0,sum6=0; int a[100][100]; scanf("%d",&n); for(i=0; i<n; i++) { for(j=0; j<3; j++) { scanf("%d",&a[i][j]); } } sum1=a[0][0]; sum2=a[0][1]; sum3=a[0][2]; sum4=a[n-1][0]; sum5=a[n-1][1]; sum6=a[n-1][2]; for(i=n-1; i>=0; i--) { for(j=n-1; j>=0; j--) { if(sum6=a[n-1][2]) { a[n-2][2]=1000; } else if(sum5=a[n-1][1]) { a[n-2][1]=1000; } else if(sum4=a[n-1][0]) { sum1+=a[i][0]; a[n-2][0]=1000; } i--; } } for(i=0; i<n; i++) { for(j=0; j<n; j++) { if(sum1=a[0][0]) { a[n+2][2]=1000; } else if(sum2=a[0][1]) { a[n+2][1]=1000; } else if(sum3=a[0][2]) { a[n+2][0]=1000; } i++; } } } */ #include <stdio.h> int grap[20][3]= {0}; int n; int calcu(int ct) { int sum=grap[0][ct]; int i, j, min=50000000;; int cot=-1; for(i=1; i<n; i++) { min=50000000; for(j=0; j<3; j++) { if(j!=ct&&min>grap[i][j]) { min=grap[i][j]; cot=j; } } sum+=min; ct=cot; cot=-1; } return sum; } int main() { int i,j,k,sum; scanf("%d",&n); for(i=0; i<n; i++) { scanf("%d %d %d",&grap[i][0],&grap[i][1],&grap[i][2]); } printf("%d\n",calcu(0)); printf("%d\n",calcu(1)); printf("%d\n",calcu(2)); }
0
0
3
minjukim3p
2020년 10월 08일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; */ /* #include <stdio.h> int main() { int n,i,j,sum,sum1=0,sum2=0,sum3=0; int a[100][100]; scanf("%d",&n); for(i=0; i<n; i++) { for(j=0; j<3; j++) { scanf("%d",&a[i][j]); } } for(i=0; i<n; i++) { for(j=0; j<3; j++) { sum+=a[i][2]; if(sum==a[i][2]){ a[i+1][2]=1000; sum=0; } sum+=a[i][0]; else if(sum==a[i][0]){ a[i+1][0]=1000; sum=0; } sum+=a[i][1]; else if(sum==a[i][1]){ a[i+1][1]=1000; sum=0; } if(a[i+1][1]==1000) { if(a[i][0]<a[i][2]){ sum3+=a[i][0]; a[i+2][0]=1000; } else{ sum3+=a[i][2]; a[i+2][2]; } } if(a[i+1][2]==1000) { if(a[i][1]<a[i][0]){ sum1+=a[i][0]; a[i+2][0]=1000; } else{ sum1+=a[i][1]; a[i+2][1]=1000; } } if(a[i+1][0]==1000) { if(a[i][1]<a[i][2]){ sum2+=a[i][2]; a[i+2][2]=1000; } else{ sum2+=a[i][1]; a[i+2][1]==1000; } i++; } } } if(sum1>=sum2&&sum2>=sum3){ printf("%d",sum3); } else if(sum2>=sum3&&sum3>=sum1){ printf("%d",sum1); } else{ printf("%d",sum2); } } */ /* #include <stdio.h> int main() { int n,i,j,sum=0,sum1=0; int a[100][100]; scanf("%d",&n); for(i=0;i<n;i++) { for(j=0;j<3;j++){ scanf("%d",&a[i][j]); } } for(i=0;i<n;i++){ for(j=0;j<n;j++){ if(a[i][0]>=a[i][2]&&a[i][1]>=a[i][2]){ sum+=a[i][2]; a[i+1][2]=1000; } else if(a[i][2]>=a[i][1]&&a[i][0]>=a[i][1]){ sum+=a[i][1]; a[i+1][1]=1000; } else if(a[i][1]>=a[i][0]&&a[i][2]>=a[i][0]){ sum+=a[i][0]; a[i+1][0]=1000; } i++; } } printf("%d",sum); } */ /* #include <Stdio.h> int grap[20][3]={0}; int n; int calcu(int ct){ int sum=grap[0][ct]; printf("%d(%d) > ",sum, grap[0][ct]); int i, j, min=50000000;; int cot=-1; for(i=1;i<n;i++){ min=50000000;; for(j=0;j<3;i++){ if(j!=ct&&min>grap[i][j]){ min=grap[i][j]; cot=j; } } sum+=min; printf("%d(%d) > ",sum,min); ct=cot; cot=-1; } return sum; } int main() { int i,j,k; scanf("%d",&n); for(i=0;i<n;i++){ scanf("%d %d %d",&grap[i][0],&grap[i][1],&grap[i][2]); } printf("%d\n",calcu(0)); printf("%d\n",calcu(1)); printf("%d\n",calcu(2)); } */
0
0
4
minjukim3p
2020년 9월 24일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ /* #include <stdio.h> int main() { int n,i,sum=0,sum1=0,sum2=0; int a[100],b[100],c[100]; scanf("%d",&n); for(i=0;i<n;i++){ scanf("%d %d %d",&a[i],&b[i],&c[i]); } if(a[0]<b[0]||a[0]<c[0]){ sum+=a[0]; a[1]=0; } else if(b[0]<a[0]||b[0]<c[0]){ sum+=b[0]; b[1]=0; } else if(c[0]<b[0]||c[0]<a[0]){ sum+=c[0]; c[1]=0; } if(a[1]==0){ if(b[1]<c[1]){ sum1+=b[1]; b[2]=0; } else if(b[1]>c[1]){ sum1+=c[1]; c[2]=0; } } else if(b[1]==0){ if(a[1]<c[1]){ sum1+=a[1]; a[2]=0; } else if(a[1]>c[1]){ sum1+=c[1]; c[2]=0; } } else if(c[1]==0){ if(a[1]<b[1]){ sum1+=a[1]; a[2]=0; } else if(a[1]>b[1]){ sum1+=b[1]; b[2]=0; } } if(a[2]==0){ if(b[2]<c[2]){ sum2+=b[2]; } else if(b[2]>c[2]){ sum2+=c[2]; } } else if(b[2]==0){ if(a[2]<c[2]){ sum2+=a[2]; } else if(a[2]>c[2]){ sum2+=c[2]; } } else if(c[2]==0){ if(a[2]<b[2]){ sum2+=a[2]; } else if(a[2]>b[2]){ sum2+=b[2]; } } printf("%d",sum+sum1+sum2); } */ /* #include <stdio.h> int main() { int n,i,j,sum=0,sum1=0; int a[100][100]; scanf("%d",&n); for(i=0;i<n;i++) { for(j=0;j<3;j++){ scanf("%d",&a[i][j]); } } for(i=0;i<n;i++){ for(j=0;j<n;j++){ if(a[i][0]>=a[i][2]&&a[i][1]>=a[i][2]){ sum+=a[i][2]; a[i+1][2]=1000; } else if(a[i][2]>=a[i][1]&&a[i][0]>=a[i][1]){ sum+=a[i][1]; a[i+1][1]=1000; } else if(a[i][1]>=a[i][0]&&a[i][2]>=a[i][0]){ sum+=a[i][0]; a[i+1][0]=1000; } i++; } } printf("%d",sum); } */ /* #include <stdio.h> int main() { int n,i,j,sum=0,sum1=0; int a[100][100]; scanf("%d",&n); for(i=0;i<n;i++) { for(j=0;j<3;j++){ scanf("%d",&a[i][j]); } } for(i=0;i<n;i++){ for(j=0;j<n;j++){ if(a[i][0]>=a[i][2]&&a[i][1]>=a[i][2]){ sum+=a[i][2]; } else if(a[i][2]>=a[i][1]&&a[i][0]>=a[i][1]){ sum+=a[i][1]; } else if(a[i][1]>=a[i][0]&&a[i][2]>=a[i][0]){ sum+=a[i][0]; } } } } */
0
0
8
minjukim3p
2020년 8월 13일
In 소스 코드 제출
/*#include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ /* #include <stdio.h> struct school { int student; int apple; }; int main() { int n,i,sum=0; struct school st[101]={0}; scanf("%d",&n); for(i=0;i<n;i++){ scanf("%d",&st[i].student); scanf("%d",&st[i].apple); sum+=st[i].apple-(st[i].apple/st[i].student*st[i].student); } printf("%d",sum); } */ /* #include <stdio.h> int rec(int n) { if(n==1){ return 1; } else if(n==2){ return 2; } return rec(n-1)+rec(n-2); } int main() { int n; scanf("%d",&n); printf("%d",rec(n)); } */ /* #include <stdio.h> int main() { int n[9],i; for(i=0;i<8;i++){ scanf("%d",&n[i]); } for(i=0;;i++){ if(n[i]==0){ break; } } } */ /* #include <stdio.h> int main() { int n,j,i,q[1000],m[1000]; scanf("%d",&n); for(i=0;i<n;i++){ scanf("%d",&m[i]); } scanf("%d",&m); for(j=0;j<m;j++){ scanf("%d",&q[j]); if(q[j]==m[i]){ printf("%d ",i); } else{ printf("-1 "); } } } */ #include <stdio.h> int main() { int n,i,j,min,sum=0,h[100][100],p_mi,mi,re_min; scanf("%d",&n); for(i=0; i<n; i++) { for(j=0; j<n; j++) { scanf("%d",&h[i][j]); } } // red start sum = h[0][0]; p_mi=0; for(i = 1; i<n ; i++) { min = 1000000; for(j=0; j<n; j++) { if(p_mi!=j &&h[i][j]<min) { min = h[i][j]; mi=j; } } p_mi=j; sum+=min; } re_min=sum; //green sum=h[0][1]; p_mi=1; sum+=min; if(re_min>sum) { re_min=sum; } //blue sum=h[0][2]; p_mi=2; sum+=min; if(re_min>sum) { re_min=sum; } printf("%d",re_min); }
0
0
3
minjukim3p
2020년 8월 06일
In 소스 코드 제출
/* #include <stdio.h> #include <stdlib.h> int main() { printf("Hello world!\n"); return 0; } */ /* #include <stdio.h> #define size 50000 int top; char stack[size]; int isfull() { if(top==size-1) { return 1; } else { return 0; } } int isempty() { if(top==-1) { return 1; } else { return 0; } } void init() { top=-1; } void push(char data) { if(isfull()) { return 0; } top++; stack[top]=data; } char pop() { char k; if(isempty()) { return 0; } k=stack[top]; stack[top]=0; top--; return k; } int main() { char str[50000]; int i; init(); scanf("%s",str); for(i=0; i<strlen(str); i++) { if(str[i]=='(') { push(str[i]); } else { pop(); } } if(str[0]==')'){ printf("bad"); } else if(isempty()) { printf("good"); } else { printf("bad"); } } */ /* #include <stdio.h> #define size 100 int top; int stack[size]; int isfull() { if(top==size-1){ return 1; } else{ return 0; } } int isempty() { if(top==-1){ return 1; } else{ return 0; } } void init() { top=-1; } void push(int data) { if(isfull()){ return 0; } top++; stack[top]=data; } int pop() { int k; if(isempty()){ return 0; } k=stack[top]; stack[top]=k; top--; return k; } void view() { for(int i = 0; i<=top; i++) { printf("%d ",stack[i]); } printf("\n"); } int main() { char str[50]; int i; init(); scanf("%s",str); for(i=0;i<strlen(str);i++){ if(str[i]=='(' || str[i]=='[') { push(str[i]); } else if(str[i]==']') { if(str[i-1]=='[') { pop(); push(3); } } else if(str[i]==')' && str[i-1]=='(') { pop(); push(2); } if(str[i]==')'){ pop(); pop(); push(2); } else if(str[i]==']'){ pop(); pop(); push(3); } if(str[i-1]=='['&&str[i+1]==']'){ push(str[i]*3); } if(str[i-1]=='('&&str[i+1]==')'){ push(str[i]*2); } if(str[i] str[i+1]) } } */ #include <Stdio.h> struct n { int student; int apple; } ; int main() { int n,i,sum; struct n st[100]; scanf("%d",&n); for(i=1;i<=n;i++){ scanf("%d",&st[i].student); scanf("%d",&st[i].apple); sum+=st[i].apple-(st[i].apple/st[i].student*st[i].student); printf("%d\n",sum); } }
0
0
2
minjukim3p
더보기
bottom of page