top of page
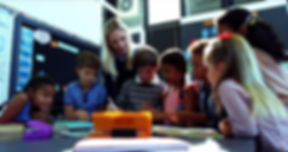
게시판 게시물
davidsdh
2020년 1월 22일
In 소스 코드 제출
//import java.util.*; // //class parents { // int a, b, c; // // parents() { // System.out.println("P1"); // } // parents(int k) { // System.out.println("P2"); // } // // void show() { // c = a+b; // } //} // //class child extends parents { // // child() { // System.out.println("C1"); // } // child(int k) { // System.out.println("C2"); // } // child(int k, char p) { // System.out.println("C3"); // } // // void show() { // c = a*b; // } //} // //class son extends child { // // // son() { // System.out.println("S1"); // } // son(int k) { // super(k, 'A'); // System.out.println("S2"); // } // void show() { // // } //} // //// upcasting , downcasting // //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // son s = new son(5); // // // } //} // //// method overloading //// method overriding //// // // // // // abstract & interface //abstract class building { // int a = 10, b, c; // // void show() { // System.out.println("Hello world"); // } // abstract void build(int a, int k); // abstract int kkk(int a); // //} // //class spca extends building { // // @Override // void build(int a, int k) { // // TODO Auto-generated method stub // // } // // @Override // int kkk(int a) { // // TODO Auto-generated method stub // return 0; // } // //} // // // //public class Main { // public static void main(String[] args) { // // } //} // interface //interface building { // final int a = 10; // // void show(); // int perple(int k); //} // //interface rawSystem { // void talk(); //} // //class spca implements building, rawSystem { // // @Override // public void show() { // // TODO Auto-generated method stub // // } // // @Override // public int perple(int k) { // // TODO Auto-generated method stub // return 0; // } // // @Override // public void talk() { // // TODO Auto-generated method stub // // } // //} // //public class Main { // public static void main(String[] args) { // // } //} /* * interface 1 > 사칙연산 * interface 2 > 제곱 * interface 3 > 루트같은 math 함수 적극 이용( sin, cos, tan ) * */ //import java.util.*; //interface arithmetic { // int sum(int a, int b); // int sub(int a, int b); // int mul(int a, int b); // int div(int a, int b); // //} //interface squared { // int pow(int a, int b); //} // //interface root { // int sqrt(int a); //} //class math implements arithmetic, squared, root { // // @Override // public int sum(int a, int b) { // // TODO Auto-generated method stub // return 0; // } // @Override // public int sub(int a, int b) { // // TODO Auto-generated method stub // return 0; // } // @Override // public int mul(int a, int b) { // // TODO Auto-generated method stub // return 0; // } // @Override // public int div(int a, int b) { // // TODO Auto-generated method stub // return 0; // } // @Override // public int sqrt(int a) { // // TODO Auto-generated method stub // return 0; // } // @Override // public int pow(int a, int b) { // // TODO Auto-generated method stub // return 0; // } // //} // // // //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // // math m = new math(); // int k = m.sum(a, b); // // // // } //}
0
0
1
davidsdh
2020년 1월 21일
In 소스 코드 제출
import java.util.*; class std { private int a; private int b; // method overloading std() { System.out.println("ccc"); } std(int n) { System.out.println("aaa"); } std(char c) { System.out.println("bbb"); } } public class Main { public static void main(String[] args) { std s = new std('A'); Arrays.sor } } import java.util.*; class math { public static void main(String[] args) { Scanner t = new Scanner(System.in); int a = t.nextInt(); int b = t.nextInt(); int c = t.nextInt(); } } package student; import java.util.*; public class school { public static void main(String[] args) { Scanner t = new Scanner(System.in); int n = t.nextInt(); int math, eng, number, sic, social, name; student sang[] = new student[n]; for(int i=0; i<n; i++) { sang[i] = new student(); } for(int i=0; i<n; i++) { System.out.print("출석번호 입력 : "); sang[i].number = t.nextInt(); System.out.print("수학점수 입력 : "); sang[i].math = t.nextInt(); System.out.print("영어점수 입력 : "); sang[i].eng = t.nextInt(); System.out.print("과학점수 입력 : "); sang[i].sic = t.nextInt(); System.out.print("사회점수 입력 : "); sang[i].social = t.nextInt(); } System.out.println("출석 수학 영어 과학 사회 평균"); for(int i=0; i<n; i++) { System.out.printf("%4d", sang[i].getNumber()); System.out.printf("%4d", sang[i].getMath()); System.out.printf("%4d",sang[i].getEng()); System.out.printf("%4d",sang[i].getSic()); System.out.printf("%4d",sang[i].getSocial()); System.out.printf("%4d\n",sang[i].getavg()); } } } package student; public class student { int math, eng, number, sic, social; public void setMath(int n) { math = n; } public void setEng(int n) { eng = n; } public void setSic(int n) { sic = n; } public void setSocial(int n) { social = n; } public void setNumber(int n) { number = n; } public int getMath() { return math; } public int getEng() { return eng; } public int getNumber() { return number; } public int getSic() { return sic; } public int getSocial() { return social; } int getavg() { return (math+eng+sic+social)/4; } } import java.util.*; class math { void math(int n, int m) { System.out.printf("%d", a+b); } void math(int n, double m) { System.out.printf("%d", a-b); } math(double n, int m) { System.out.printf("%d", a*b); } math(double n , double m) { System.out.printf("%d", a%b); } public static void main(String[] args) { Scanner t = new Scanner(System.in); int a = t.nextInt(); double b = t.nextDouble(); math m = new math(a, b); } }
0
0
1
davidsdh
2020년 1월 15일
In 소스 코드 제출
//package Car; // //class car { // // public int Speed; // public int direction; // // public void Turndrect(int n) { // direction = direction + n; // } // // public int getdirection() { // return direction; // } // public int setSpeed(int n) { // Speed = n; // return 0; // } // // int getSpeed() { // return Speed; // } // //} // // //public class Main { // // public static void main(String[] args) { // // TODO Auto-generated method stub // // car car1 = new car(); // // car1.setSpeed(80); // // System.out.println(car1.getSpeed()); // // } // //} package school; public class impormaion { public int Student; public setStudent(); Student = 10109; return 0; } // // //import java.util.*; // //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // // int n = t.nextInt(); // // int map[][] = new int[n][n]; // int k =1; // for(int i=0; i<n; i++) { // for(int j=0; j<n; j++) { // map[i][j] = k++; // } // } // // for(int i=0; i<n; i++) { // if(i%2==0) { // for(int j=0; j<n; j++) { // System.out.print(map[i][j]+" "); // } // } // else { // for(int j=n-1; j>=0; j--) { // System.out.print(map[i][j]+" "); // } // } // System.out.println(); // } // } //} // //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int map[][] = new int[11][10]; // int k = 1; // for(int i=0; i<11; i++) { // for(int j=0; j<10; j++) { // map[i][j] = t.nextInt(); // } // } // for(int j=0; j<10; j++) { // int a=0; // if(map[10][j]==1) { // for(int i=9; i>=0; i--) { // if(map[i][j] > 0) { // a++; // break; // } // else if(map[i][j] < 0) { // a--; // break; // } // else a=0; // // } // if(a==0) // System.out.println((j+1) + " safe"); // else if (a<0) // System.out.println((j+1) + " fall"); // else // System.out.println((j+1) + " crash"); // // } // else System.out.print(""); // // } // // // // } //} // //import java.util.*; //public class Main { // public static void main(String[] args) { // int c; // c= Math.max(10, 20); // System.out.println(c); // // } //}
0
0
3
davidsdh
2020년 1월 14일
In 소스 코드 제출
//import java.util.*; //public class Main { // public static void main(String[] args) { // int j, i; // int x, y, n; // int arr[][] = new int[20][20]; // Scanner t = new Scanner(System.in); // for(i=1; i<=19; i++) { // for(j=1; j<=19; j++) { // arr[i][j] = t.nextInt(); // } // } // n = t.nextInt(); // for(i=1; i<=n; i++) { // x = t.nextInt(); // y =t.nextInt(); // for(j=1; j<=19; j++) { // if(arr[x][j]==0) // arr[x][j]=1; // else arr[x][j]=0; // } // for(j=0; j<=19; j++) { // if(arr[j][y]==0) arr[j][y]=1; // else arr[j][y]=0; // } // } // for(i=1; i<=19; i++) { // for(j=1; j<=19; j++) { // System.out.printf("%d ", arr[i][j]); // } // System.out.println(); // } // } //} // System.out.printf("%d(%d,%d)\t", map[i][j], i, j); //import java.util.*; //public class Main { // public static void main(String[] args) { // //i가 세로 j가 가로 // Scanner t = new Scanner(System.in); // int n = t.nextInt(); // int map[][] = new int[n][n]; // // int k = 1; // for(int i=0; i<n; i++) { // for(int j=0; j<n; j++) { // map[i][j] = k++; // } // } // for(int i=0; i<n; i++) { // for(int j=0; j<n; j++) { // System.out.printf("%d ", map[i][j]); // } // System.out.println(); // } // // // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int n = t.nextInt(); // int map[][] = new int[n][n]; // // int k = 1; // for(int i=0; i<n; i++) { // for(int j=0; j<n; j++) { // map[i][j] = k++; // } // } // for(int i=0; i<n; i++) { // for(int j=n-1; j>=0; j--) { // System.out.printf("%d ", map[i][j]); // } // System.out.println(); // } // // // } //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int n = t.nextInt(); // int map[][] = new int[n][n]; // //i가 세로 j가 가로 // int k = 1; // for(int i=0; i<n; i++) { // for(int j=0; j<n; j++) { // map[i][j] = k++; // } // } // for(int i=0; i<n; i++) { // for(int j=0; j<n; j++) { // System.out.printf("%d ", map[j][i]); // } // System.out.println(); // } // } // // } //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int n = t.nextInt(); // int map[][]= new int[n][n]; // int k = 1; // for(int i=0; i<n; i++) { // for(int j=0; j<n; j++) { // map[i][j] = k++; // } // } // for(int i=n-1; i>=0; i--) { // for(int j=0; j<n; j++) { // System.out.printf("%d ", map[j][i]); // } // System.out.println(); // } // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int n = t.nextInt(); //n은 세로 // int m = t.nextInt(); //m은 가로 // int map[][] = new int[n][m]; // int k = 1; // for(int i=0; i<n; i++) { // for(int j=0; j<m; j++) { // map[i][j] = k++; // } // } // for(int i=n-1; i>=0; i--) { // for(int j=m-1; j>=0; j--) { // System.out.printf("%d ", map[i][j]); // } // System.out.println(); // } // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int n = t.nextInt(); // int m = t.nextInt(); // int map[][] =new int[n][m]; // int k=1; // for(int i=0; i<n; i++) { // for(int j=0; j<m; j++) { // map[i][j] = k++; // } // } // for(int i=n-1; i>=0; i--) { // for(int j=0; j<m; j++) { // System.out.printf("%d ", map[i][j]); // } // System.out.println(); // } // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int n = t.nextInt(); // int m = t.nextInt(); // int map[][] = new int[n][m]; // int k=1; // for(int j=0; j<m; j++) { // for(int i=n-1; i>=0; i--) { // map[i][j]= k++; // } // } // for(int i=0; i<n; i++) { // for(int j=m-1; j>=0; j--) { // System.out.print(map[i][j] +" "); // } // System.out.println(); // } // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int n = t.nextInt(); // int m = t.nextInt(); // int map[][] = new int[n][m]; // int k=1; // for(int j=0; j<m; j++) { // for(int i=0; i<n; i++) { // map[i][j]= k++; // } // } // for(int i=0; i<n; i++) { // for(int j=m-1; j>=0; j--) { // System.out.printf("%d ", map[i][j]); // } // System.out.println(); // } // } //}
0
0
1
davidsdh
2020년 1월 08일
In 소스 코드 제출
//import java.util.*; //public class Main { //public static void main(String[] args) { // // Scanner t =new Scanner(System.in); // int a = t.nextInt(); // int arr[] = new int[a]; // // for(int i=0; i < a; i++) // { // arr[i] = t.nextInt(); // } // // for(int i=0; i < a; i++) { // for(int j = i; j < i+a; j++) { // System.out.printf("%d ", arr[j%(a)]); // } // System.out.println(); // } // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t =new Scanner(System.in); // String str = t.next(); // int cnt1=0, cnt2=0; // // for(int i=0; i<str.length(); i++) { // if(str.charAt(i)=='(') { // cnt1++; // } // else if(str.charAt(i)==')') { // cnt2++; // } // } // System.out.printf("%d %d", cnt1, cnt2); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int arr[] = new int[a]; // // for(int i=0; i < a; i++) { // arr[i] = t.nextInt(); // } // for (int i = 0; i < a; i++) // { // System.out.printf("%d : ", i + 1); // for (int j = 0; j < a; j++) { // if (i == j); // else if (arr[i] < arr[j]) // System.out.printf(" < "); // else if (arr[j] < arr[i]) // System.out.printf(" > "); // else if (arr[i] == arr[j]) // System.out.printf(" = "); // } // System.out.println(); // } // } //}
0
0
1
davidsdh
2020년 1월 07일
In 소스 코드 제출
//import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int h = t.nextInt(); // int k = t.nextInt(); // int i, j; // char d = t.next().charAt(0); // for(i=0; i<h; i++) { // if(d=='L') // for(j=0; j<i; j++) // System.out.print(" "); // // else // for(j=h-i-1; j>0; j--) // System.out.print(" "); // for (j=0; j<k; j++) { // System.out.print("*"); // } // if(!(i==k)) // System.out.println(); // } // // } // // } // //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int r = t.nextInt(); // int g = t.nextInt(); // int b = t.nextInt(); // int i, j, k, c=0; // for(i=0; i<r; i++) { // for(j=0; j<g; j++) { // for(k=0; k<b; k++) { // System.out.printf("%d %d %d\n", i, j, k); // c++; // } // } // } // System.out.printf("%d", c); // // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int sum = 0; // int i, j; // for (i = 1; i <= a; i++) { // for (j = 1; j <= i; j++) { // sum += j; // } // } // System.out.printf("%d", sum); // // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int m = t.nextInt(); // int g = t.nextInt(); // int i; // if((m%5)==0) // i=((90-m)/5); // else // i=((90-m)/5)+1; // // System.out.printf("%d", g+i); // // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // int c = t.nextInt(); // if(b>c&&a>=c) // System.out.printf("%d", a); // else if(a>b&&b>=c) // System.out.printf("%d", b); // else System.out.printf("%d", c); // } //} //import java.util.*; // //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // // int a = t.nextInt(); // int b = t.nextInt(); // int c = t.nextInt(); // // if(a>b) { // int temp = a; // a = b; // b = temp; // } // if(b>c) { // int temp = b; // b = c; // c = temp; // } // if(a>b) { // System.out.printf("%d", a); // } // else { // System.out.printf("%d", c); // // } // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b, c; // // b=a%10; // a=a/10; // c=((b*10)+a)*2; // if(c>=100) // c=c-100; // System.out.printf("%d\n", c); // if(c>50) { // System.out.println("OH MY GOD"); // } // else System.out.println("GOOD"); // } //} //import java.util.*; //백준 //public class Main { // public static void main(String args[]) { // Scanner sc = new Scanner(System.in); // String[] eight = {"000","001","010","011","100","101","110","111"}; // String s = sc.nextLine(); // boolean start = true; // if (s.length() == 1 && s.charAt(0) == '0') { // System.out.print(0); // } // for (int i=0; i<s.length(); i++) { // int n = s.charAt(i) - '0'; // if (start == true && n < 4) { // if (n == 0) { // continue; // } else if (n == 1) { // System.out.print("1"); // } else if (n == 2) { // System.out.print("10"); // } else if (n == 3) { // System.out.print("11"); // } // start = false; // } else { // System.out.print(eight[n]); // start = false; // } // } // System.out.println(); // } //} //import java.util.*; //public class Main{ // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int h = t.nextInt(); // int m = t.nextInt(); // if(m>=30) // System.out.printf("%d %d", h, m-30); // else if (h==0&&m<30) // System.out.printf("%d %d", h+23, m+30); // else // System.out.printf("%d %d", h-1, m+30); // } //} //
0
0
2
davidsdh
2020년 1월 05일
In 소스 코드 제출
//import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // if((!(a==true)&&b)||(a&&!b)) // System.out.println("1"); // else // System.out.println("0"); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // System.out.printf("%d", a!=b? 1:0); // //} //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // if(a==b) // System.out.println("1"); // else // System.out.println("0"); // } //} //import java.util.*; // //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // // int a = t.nextInt(); // int b = t.nextInt(); // boolean x, y; // if(a==1) x = true; // else x = false; // // System.out.println(x&&x); // // } //} //import java.math.BigInteger; //import java.util.*; // //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // // BigInteger a = t.nextBigInteger(); // // System.out.println(a); // // // } //} //import java.util.*; //public class Main{ // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // double a = t.nextDouble(); // double b = t.nextDouble(); // if(a<150) { // a=a-100;} // else if(a>=150&&a<160) { // a=(a-150)/2+50; } // else { // a=(a-100)*0.9; // } // b=(b-a)*100/a; // if(b<=10) // System.out.println("정상"); // else if (b<=20) // System.out.println("과체중"); // else // System.out.println("비만"); // // // // } //} // //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // if (a>0) // System.out.println("양수"); // else if (a==0) // System.out.println("0"); // else // System.out.println("음수"); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // int c = t.nextInt(); // int d = t.nextInt(); // // switch(a+b+c+d) { // case 0: // System.out.println("모"); // break; // case 1: // System.out.println("도"); // break; // case 2: // System.out.println("개"); // break; // case 3: // System.out.println("걸"); // break; // case 4: // System.out.println("윷"); // break; // // } // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // int i, n; // for (i=1; i<=a; i++) { // for(n=1; n<=a; n++ ) { // if(i==1||i==a||n==1||n==a||(i+n-1)%b==0) // System.out.print("*"); // else // System.out.print(" "); // } // System.out.println(); // } // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // int i, j, k; // for(k=1; k<=b; k++) { // for(i=1; i<=a; i++) { // for(j=1; j<i; j++) { // System.out.print(" "); // } // System.out.println("*"); // } // // for(i=a-1; i>=1; i--) { // for(j=1; j<i; j++) { // System.out.print(" "); // } // System.out.println("*"); // } // // } // } //}
0
0
3
davidsdh
2019년 12월 31일
In 소스 코드 제출
//public class Main { // public static void main(String[] args) { // System.out.println("\"!@#$%^&*()\""); // } //} //public class Main { // public static void main(String[] args) { // System.out.println("'Hello'"); // } //} //public class Main { // public static void main(String[] args) { // System.out.println("\"C:\\Download\\hello.cpp\""); // } //} //public class Main { // public static void main(String[] args) { // System.out.println("\"Hello World\""); // } //} //public class Main { // public static void main(String[] args) { // System.out.println("Hello\nWorld"); // } //} //public class Main { // public static void main(String[] args) { // System.out.println("Hello World"); // } //} //public class Main { // public static void main(String[] args) { // System.out.println("Hello"); // } //} //public class Main { // public static void main(String[] args) { // // System.out.println(); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // System.out.println(a); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // char n = t.next().charAt(0); // System.out.println(n); // // // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // double a = t.nextDouble(); // System.out.format("%f", a); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a= t.nextInt(); // int b= t.nextInt(); // System.out.println(a+" "+ b); // //System.out.printf("%d %d", a ,b); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // char a = t.next().charAt(0); // char b = t.next().charAt(0); // System.out.println(b+" "+ a); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // double a = t.nextDouble(); // System.out.printf("%.2f", a); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // System.out.println(a+" "+a+" "+a); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // System.out.println(a/b); // // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // int c = t.nextInt(); // System.out.printf("%d\n%.1f", a+b+c, (double)(a+b+c)/3); // } //} //import java.util.*; //public class Main { // public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int a = t.nextInt(); // int b = t.nextInt(); // int c = t.nextInt(); // if(a%2==0) { // // } // } //} import java.util.*; public class Main { public static void main(String[] args) { Scanner t = new Scanner(System.in); int a = t.nextInt(); int b = t.nextInt(); int c = t.nextInt(); if (a+b<=c) System.out.println("삼각형아님"); else if (a==b&&a==c) System.out.println("정삼각형"); else if(a==b||b==c||a==c) System.out.println("이등변삼각형"); else if(a*a+b*b==c*c) System.out.println("직각삼각형"); else System.out.println("삼각형"); } }
0
0
2
davidsdh
더보기
bottom of page