# import matplotlib.pyplot as plt# import numpy as np## x = np.linspace(-10,10,100)# y= x**3## plt.plot (x,y)# plt.xscale('symlog')## plt.show()# import matplotlib.pyplot as plt# import numpy as np## x=np.linspace (0,5,100)# y=np.exp(x)## plt.plot(x,y)# plt.yscale('log')## plt.show()# import matplotlib.pyplot as plt# import numpy as np## x = np.arange(0, 2, 0.2)# y= np.arange(0, 6, 0.5)## plt.plot (y, y, 'r--', y, y**2, 'bo', y,y**3,'g-.')# plt.axis ([0,6,0,20])# plt.xlabel ('X-Axis', labelpad=20, fontdict={'family':'serif'},loc='right')# plt.show()# import matplotlib.pyplot as plt# import numpy as np## x=np.arange(0, 2, 0.2)## plt.plot(x,x,'r-')# plt.plot(x,x**2,'bo', linewidth = 2)# plt.grid(True, axis = 'y', color = 'pink', alpha=0.5, linestyle='-.')# plt.axvline (1,0.2,0.3, color='pink', linewidth=2)# plt.vlines(1.75, 2, 6, color='green',linewidth = 4)# plt.xticks([0,0.5,1,1.5,2])# plt.yticks(np.arange(1,6))# title_right = plt.title('Graph Title', loc='right', pad=20)## title_font = {# 'fontsize': 16,# 'fontweight': 'bold'# }# title_left = plt.title('Graph Title', fontdict=title_font, loc='left', pad=20)## print(title_left.get_position())# print(title_left.get_text())## print(title_right.get_position())# print(title_right.get_text())# ## # plt.show()## import matplotlib.pyplot as plt# import numpy as np## x=np.arange(5)# years = ['2018', '2019', '2020', '2021', '2022']# values = [20, 220, 448, 397, 500]# colors = ['y', 'dodgerblue','C2']## plt.bar(x, values, color=colors, width= 0.4, align='edge', tick_label=years)# plt.xticks(x, years)# plt.xlabel('Year', labelpad = 15, color='blue', size=10, loc='center')# plt.ylabel('# of students', labelpad=15, color='blue', size=10, loc='center')## plt.show()## #plt.barh 랑 plt.yticks 사용하고 width 대신 height 를 사용한다## #SCATTER PLOT## import matplotlib.pyplot as plt# import numpy as np## np.random.seed(0)## n = 50# x= np.random.rand(n)# y= np.random.rand(n)# area = (30 * np.random.rand(n))**2# colors = np.random.rand(n)## plt.scatter (x, y, s=area, c=colors, cmap='Spectral')# plt.colorbar()# plt.show()#3d# from mpl_toolkits.mplot3d import Axes3D# import matplotlib.pyplot as plt# import numpy as np## n=100# xmin, xmax, ymin, ymax, zmin, zmax = 0,20,0,20,0,50# cmin, cmax = 0, 2## xs = np.array([(xmax - xmin)*np.random.random_sample() + xmin for i in range(n)])# ys = np.array([(ymax - ymin)*np.random.random_sample() + ymin for i in range (n)])# zs = np.array([(zmax - zmin)*np.random.random_sample() + zmin for i in range(n)])# color = np.array([(cmax - cmin)*np.random.random_sample() + cmin for i in range(n)])## fig = plt.figure(figsize=(6, 6))# ax = fig.add_subplot (111, projection = '3d')# ax.scatter(xs, ys, zs, c=color, marker='*', s=15, cmap='Greens')## plt.show()# import matplotlib.pyplot as plt# weight = [68,81,64,56,78,74,61,62,65,70,69,70,77,66,68,59,72,80,59,67,81,57,76,78,76]# plt.hist(weight, label = 'bins=10')# plt.hist(weight, bins=30, label = 'bins=30')# plt.legend()# plt.show()import matplotlib.pyplot as pltdata = []f = open("./data.txt", 'r', encoding='UTF8')while True: line = f.readline() if not line: break line = line.split('\t') data.append(line)f.close()for i in range(len(data)): for j in range(len(data[i])): print(data[i][j], end=' ') print()import matplotlib.pyplot as plt# for j in range(3, 7):# vData = []# for i in range(1, len(data[j])):# if data[j][i] == '-':# vData.append(0)# else:# vData.append(int(data[j][i]))## print(vData)adata = []for i in range(2, len(data[3])): if data [3][i] == '-': adata.append(0) else: adata.append(int(data[3][i]))bdata = []for i in range (2, len(data[1])): bdata.append(data[1][i])print(adata)print(bdata)import matplotlib.pyplot as pltimport numpy as npx = np.arange (15)types = [(bdata)]values = [(adata)]plt.bar(x, values)plt.xticks(x, types)plt.show()
top of page
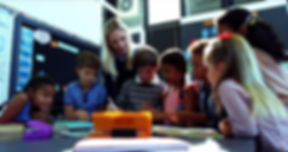
실제 작동 상태를 확인하려면 라이브 사이트로 이동하세요.
20230621
20230621
댓글 0개
좋아요
댓글(0)
bottom of page